Code for N, Support for 3
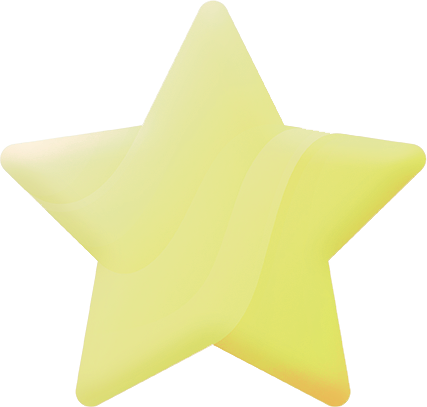
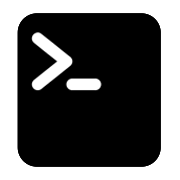
Code for N, Support for 3
In product development, it's tempting to build features that only support exactly what’s needed today. But what if you wrote your code to handle more than that—say, n things—while letting the app or API enforce temporary limits, like 3?
That’s not overengineering. That’s just engineering--designing for extensibility.
I call this pattern Code for N, Support for 3.
What Is “Code for N, Support for 3”?
It’s a development approach where:
- The core logic is general and can handle many cases (e.g., unlimited tags, roles, permissions).
- Product constraints (like "only 3 allowed") are applied at the edges—in the frontend UI or at the API level.
- The logic is ready to scale or adapt, without rewriting the core system.
Think of it like building a restaurant kitchen that can make 50 dishes, even if the menu only lists 5 today.
Example: Permissions System
Let’s say you're building role-based access control. The product team only needs:
- Admin
- Editor
- Viewer
You could write:
if (user.role === 'admin') {
// do admin stuff
}
But with Code for N, you build a general system:
- Define structured roles and permissions.
- Create a matrix of capabilities:
canManageUsers(user)
,canEditPosts(user)
, etc. - Store role-permission mappings in a config or database.
Then, apply constraints like “only use these 3 roles” in the admin panel or frontend. Not in the logic itself.
Now, when the product team says “we need a Contributor role,” you’re already ready.
Why This Pattern Works
-
Extensibility isn't overengineering
You solve the general case, but only expose the subset that’s needed now. -
Better separation of concerns
Your logic handles what’s possible. The app or API handles what’s permissible. -
Testability and maintainability
General code is more modular and easier to unit test. -
Avoids technical debt
You won’t have to undo shortcut conditionals later when requirements grow.
When to Use This Pattern
You should aim to use Code for N, Support for 3 whenever possible—especially when you can see even a hint of future variation coming. It keeps your code clean, adaptable, and makes you look like a genius six months later.
That said, sometimes you will have hardcoded a few cases up front, especially if:
- The constraint feels truly permanent (like only supporting one language).
- You’re in a rush and just need something to work.
But here's the rule of thumb:
If something repeats three times, it’s time to generalize.
Don’t let one-off hacks pile up. Use the repetition as your signal to refactor toward extensibility.
Naming It
I like the phrase “Code for N, Support for 3” because it’s memorable and speaks to the core idea. But it could also be referenced in principle as:
- Design for Extensibility
- Separation of Concerns
- Open/Closed Principle (SOLID)
- Constraint-Late Design
In short, build the engine, not just the car — enable the product team choose the roads, the route, and the destination, and adapt as needed.