Unwrapping optionals in Swift
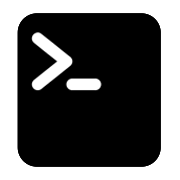
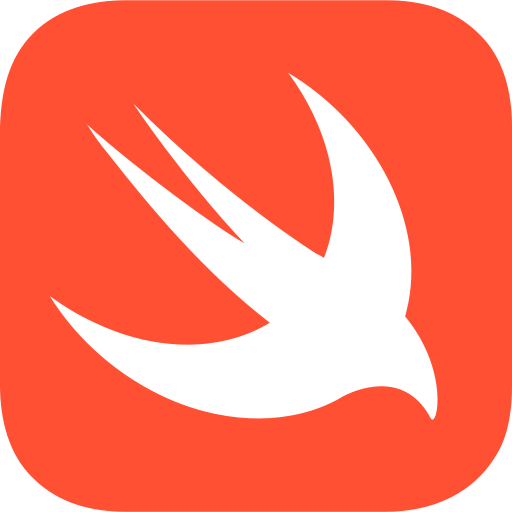
Swift brings a lot of safety features to iOS development. One such feature is the concept of Optionals. An Optional in Swift represents a variable that can hold either a value or no value at all (nil).
In many cases, you might need to extract the actual value from an optional, which is known as 'unwrapping'. This blog post will walk you through the basics of unwrapping optionals in Swift.
1. Force unwrapping
This is the simplest way to unwrap an optional, but it's also the riskiest. You use an exclamation mark (!) right after the optional.
let name: String? = "John McGlone"
let unwrappedName = name!
print(unwrappedName) // Outputs: John McGlone
But be cautious! If the optional has no value (is nil) and you try to force unwrap it, your app will crash.
let name: String!
let unwrappedName = name! // This crashes the app
print(unwrappedName)
2. Optional Binding
This is a safer way to unwrap an optional. You use the if let or guard let syntax.
let age: Int? = 35
if let unwrappedAge = age {
print("John's age is \(unwrappedAge)")
} else {
print("No value found")
}
3. Nil Coalescing Operator
This operator allows you to provide a default value in case the optional is nil.
let nickname: String? = nil
let actualName = nickname ?? "John"
print(actualName) // Outputs: John
let nickname: String? = "JP"
let actualName = nickname ?? "John"
print(actualName) // Outputs: JP
4. Optional Chaining
If you want to call properties, methods, or subscripts on an optional that might be nil, you can use optional chaining.
let person: Person? = getPerson()
let age = person?.getAge()
Here, age will also be an optional because the entire chain becomes optional if any part is nil.
Conclusion
Swift's optionals provide a robust way to deal with the absence of a value. While there are multiple ways to unwrap these optionals, you should select the right method depending on the context to ensure your app remains crash-free, efficient, and your code remains clean.
Always prefer safe unwrapping methods like optional binding over riskier approaches like forced unwrapping.