Why Your App Should Be API-Driven
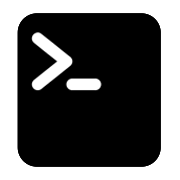
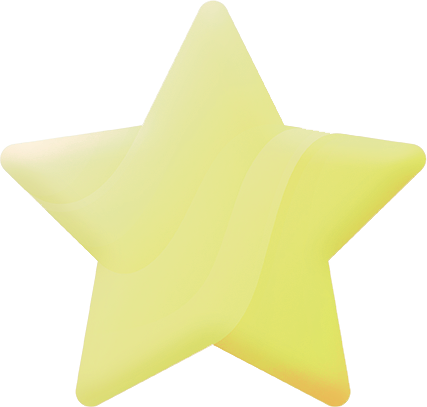
When building an app—web, mobile, desktop, whatever—there’s a core architectural choice that impacts everything: how you define the boundary between frontend and backend.
There are three common approaches:
- Backend-driven: the API mirrors the backend’s internal structure.
- Frontend-driven: the API serves exactly what the frontend needs.
- API-driven: the API is treated as a standalone product with a clean, platform-agnostic contract.
Let’s break these down—and see why API-driven is the one you actually want.
1. Backend-Driven APIs Reflect Your Internal Mess
When backend engineers lead API design, the endpoints often just mirror the database or internal architecture.
Example:
You might get endpoints like:
GET /users/123/profile-fields
GET /users/123/settings-nodes
GET /roles-by-user-id?id=123
These make perfect sense to the backend—they follow the data structure. But for the frontend (or a mobile app, or CLI), they’re a mess. You end up needing three separate calls just to render one screen.
Backend-driven APIs make integration harder and tie your client experience to internal implementation details. Not good.
2. Frontend-Driven APIs Are Better—But Still Limited
Frontend developers are the actual consumers of your API, so naturally they know what data they need and how it should be shaped. This leads to APIs that better serve real UI needs.
Example:
Instead of five endpoints to stitch together a profile, the frontend might request:
GET /api/user-dashboard
And get back everything it needs for the initial render.
This is great for user experience and development speed. But if the API is only designed around one frontend, it risks becoming tightly coupled to that platform.
Need to build a CLI later? Good luck reusing /api/user-dashboard
if it’s shaped like a mobile screen.
3. API-Driven Architecture Is the Sweet Spot
Instead of being an extension of your backend or shaped just for your frontend, an API-driven approach treats the API as its own product.
It becomes the shared language between all platforms. It's simple, consistent, and platform-agnostic.
Example:
Imagine you build an app where users can manage tasks. Your API might look like:
GET /api/tasks
POST /api/tasks
PATCH /api/tasks/:id
That works beautifully for your web app, your mobile app, and your CLI. Each one can fetch or mutate data in a predictable way using a common protocol—usually JSON over HTTP.
Now your frontend can use these APIs cleanly. Your backend doesn’t expose its guts. And future platforms won’t require rewrites or weird workarounds.
TL;DR
- Backend-driven APIs leak internal structure and are hard to use.
- Frontend-driven APIs are better—but risk being too tailored to a single platform.
- API-driven means designing clean, reusable contracts that serve all platforms well.
Think of it like this: frontend is to user interface as API is to developer interface. You wouldn’t let the database team design your UI. Don’t let them design your API either.
Design your API like a product, and everything else gets easier.